API testing is software testing that focuses on checking the "application programming interface," or API. API testing makes it possible to start testing early in the development cycle, before the user interface is ready, which brings significant benefits when it comes to the cost of bug fixing and the speed of application UI development. In the following article, we will focus on the more specific goals of such testing, discuss the types of APIs currently in use, and discuss the most popular applications for automating them.
APIS
An API is a set of rules, protocols and tools that allow different applications or services to communicate with each other, allowing within one service/application to access the functionality of other applications or services - for example, in an email client we can display the weather forecast, or on a restaurant website we can see a map snippet from Google Maps showing directions to the restaurant. APIs are widely used in the software and application and service development industry, allowing integration between different systems (microservices), extending functionality by using external services, and creating cross-platform applications.
API testing
In the pyramid of tests, API tests are placed at lower levels - they are usually classified as integration tests, since they focus on testing the API and communication between the application and external services or application modules. In practice, API tests can range from unit tests at the level of individual API endpoints to end-to-end tests that use the API as part of a broader test scenario.
Depending on the organization and the project, the responsibility for creating API tests may be carried by someone with a different role on the team. This could be a tester, a developer, or even DevOps. It is important that those responsible for creating API tests have both technical knowledge of APIs and software testing skills.
API testing objectives
During testing, the designated person uses tools to send queries to the API and analyze the responses. This allows checking such aspects as:
- Correctness of operation and functionality - API testing allows you to verify that the API works as expected. You can verify whether API responses are correct or response statuses are legitimate, etc.
- Security, especially authorization and authentication- API tests verify that authorization and authentication mechanisms work as required, i.e. whether API access is properly controlled.
- Performance and stability assurance-App API tests assess API performance by measuring response time, throughput or load to verify that the API is running smoothly and as expected. In addition, regular testing identifies potential problems early, making it easier to fix them before making changes in production.
- Integration and interactions- API tests are particularly useful when integrating external services or applications. They allow you to verify that interactions with other systems are correct and as expected.
Automation of API tests
API tests can be performed manually, but in order to speed up the process, achieve repeatability and reduce the need to devote human resources, it is worth automating them. An additional advantage of automation is to facilitate integration with CI/CD (Continuous Integration/Continuous Deployment) processes.
There are many tools available on the market for API testing, but we should always start our selection by analyzing the preferences and needs of the team, as well as the type of API to be tested.
API types
It is worth remembering that there are several types of APIs, which differ in the way they communicate, protocols, technologies used and functionalities:
-
RESTful API, or simply REST (Representational State Transfer), is an architectural style that defines the rules of communication between client and server. A RESTful API (or REST API) uses rules such as the use of standard HTTP methods (GET, POST, PUT, DELETE), URIs (Uniform Resource Identifiers) and data formats such as JSON or XML
-
The SOAP (Simple Object Access Protocol) API is a communication protocol that enables data exchange between applications in the form of XML messages. The SOAP API defines the structure and format of communication, and the client and server communicate using special SOAP messages.
-
The GraphQL API, which is a query language and query execution environment that allows flexible queries to be made to the API to get exactly the data the client needs. This represents a natural evolution from the REST API, where the client accepts data with a predetermined shape. GraphQL queries are declarative, while API responses are in JSON format.
API test automation tools
Listed below are some popular tools that are often used for API testing:
-
Postman, is one of the most popular API testing tools. It has an intuitive interface that allows you to send HTTP requests, analyze responses, create test scripts, manage environments and generate reports.
-
Swagger (OpenAPI), is a framework that allows you to create API documentation in OpenAPI format. In addition, Swagger provides tools for testing APIs against defined specifications. It allows you to send queries, check schemas, explore APIs and generate client code.
-
curl, is a command-line tool available for various platforms that allows sending HTTP/HTTPS requests. Curl is a flexible tool that can be used for simple API testing by manually creating queries and analyzing responses.
-
JMeter, is a performance and load testing tool, but can also be used for API testing. It allows you to send multiple requests simultaneously, monitor response times, generate reports and simulate load on the API.
-
SoapUI, is a tool that specializes in testing web services, including SOAP-based APIs. It allows sending requests, verifying responses, testing multiple scenarios and automating tests.
-
RestAssured, is a popular tool for developers that makes it easy to write API tests in Java. It provides a simple interface for sending requests, analyzing responses and validating data.
Postman
Postman has become a de facto standard tool when it comes to REST API testing - in addition to the basic mechanisms, it offers several specific features that greatly streamline the entire process:
- Create and send requests- Postman allows you to create different types of HTTP requests, such as GET, POST, PUT, DELETE, PATCH, etc. You can customize headers, parameters, request content and data format, such as JSON or XML. It also allows you to set authorization and authentication if required by the API.
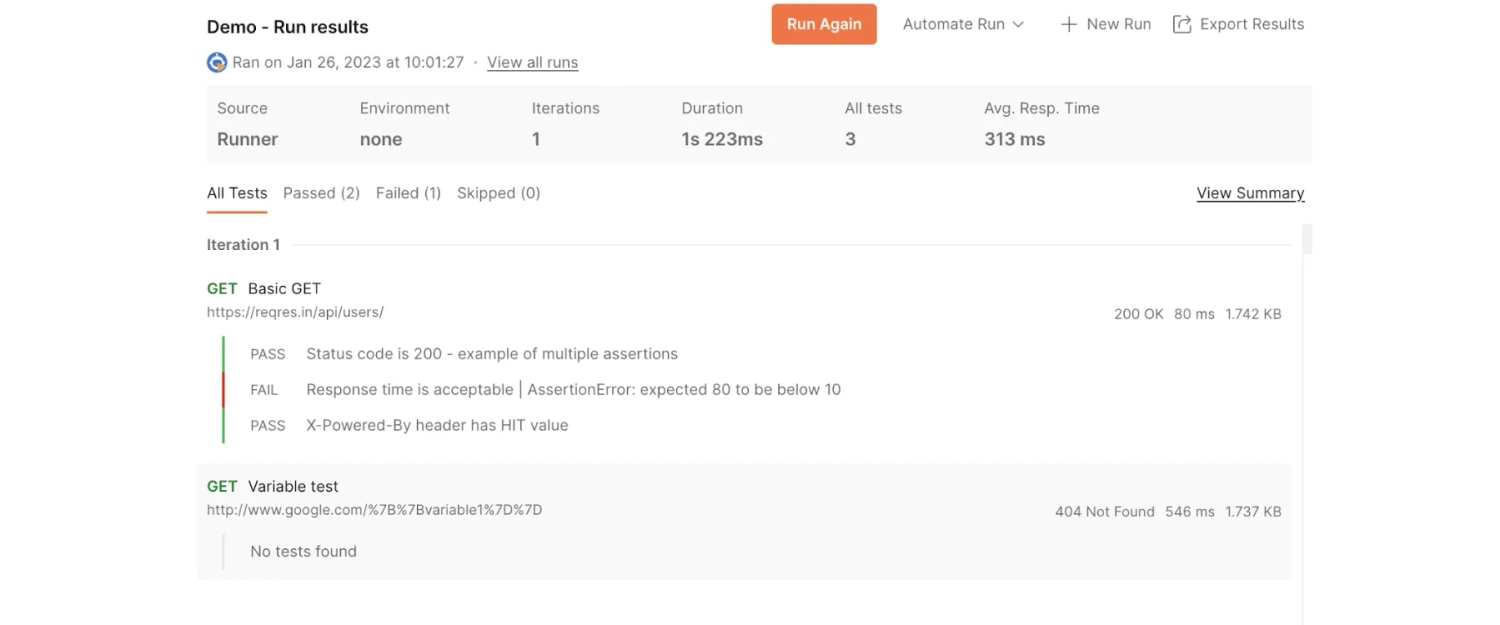
- Environments and managing them- Postman allows you to create and manage different environments that make it easier to test the API in different configurations. You can define environment variables, such as URLs, access keys, etc., which can be easily accessed and make it easy to change between environments.
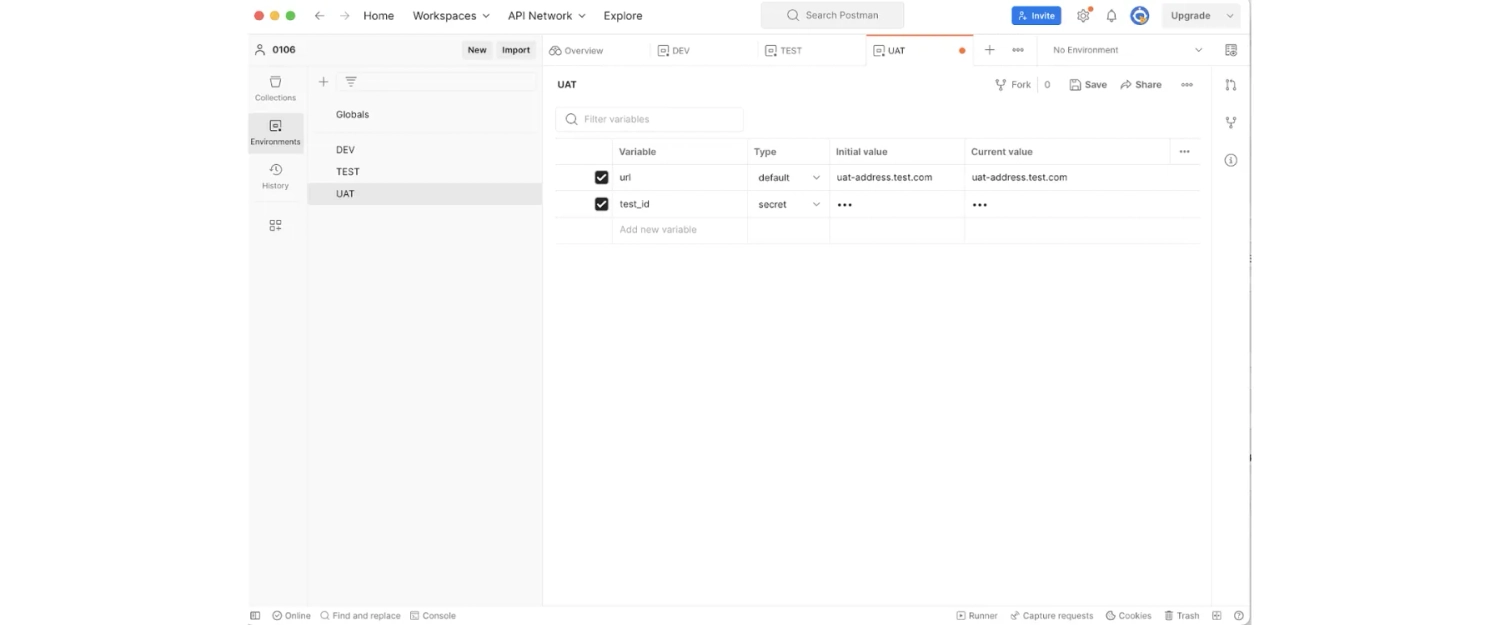
- Creating test scripts- Postman offers the ability to create test scripts to automate API testing. Test scripts are based on JavaScript and the ChaiAssertionLibrary and allow, among other things, to verify API responses, check data, perform assertions, handle logical conditions, for example:
// Checks the response status code // Checks the response status code pm.test("Status code is 200", function () { pm.response.to.have.status(200); }); // Checks if the response contains expected data pm.test("Response body contains expected data", function () { var jsonData = pm.response.json(); pm.expect(jsonData.cars.length).to.be.above(0); pm.expect(jsonData.cars[0].name).to.equal("BMW X3"); });
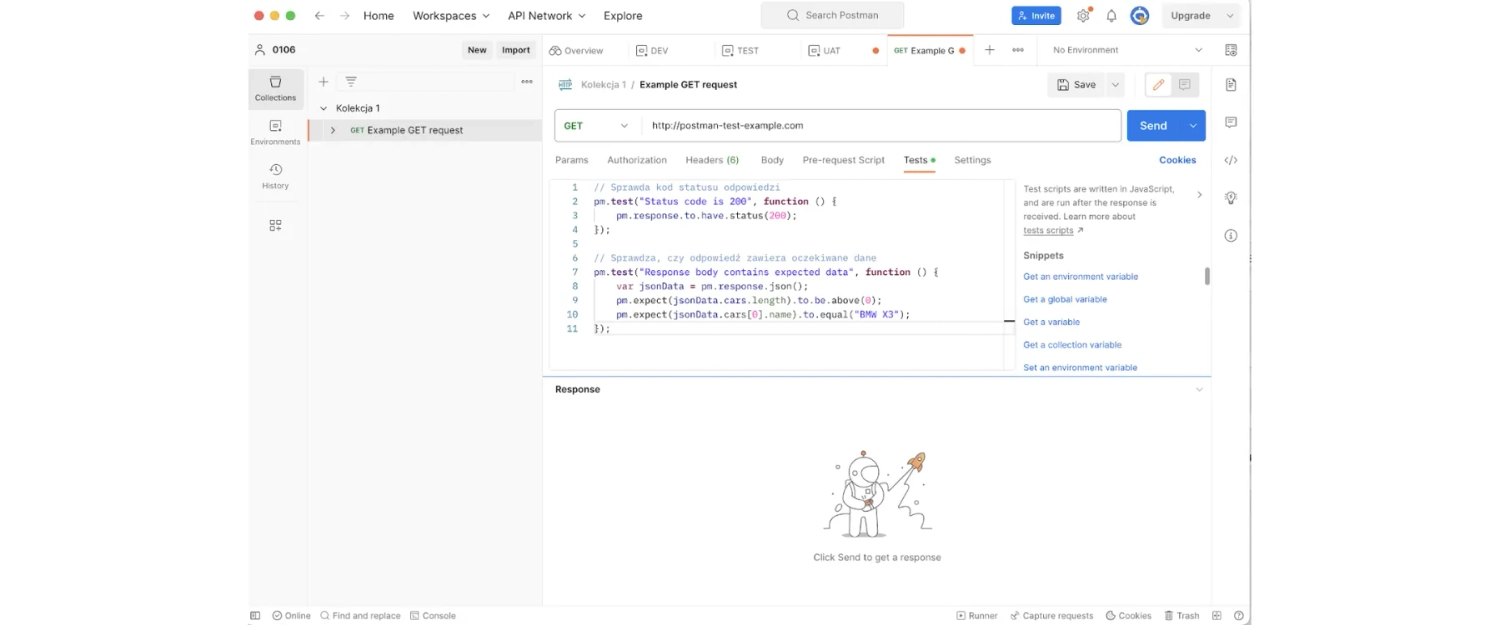
- Generate API documentation- Postman allows you to generate API documentation based on defined queries. You can share the documentation of your API with other team members or customers, so they can easily use the API and understand the available features.
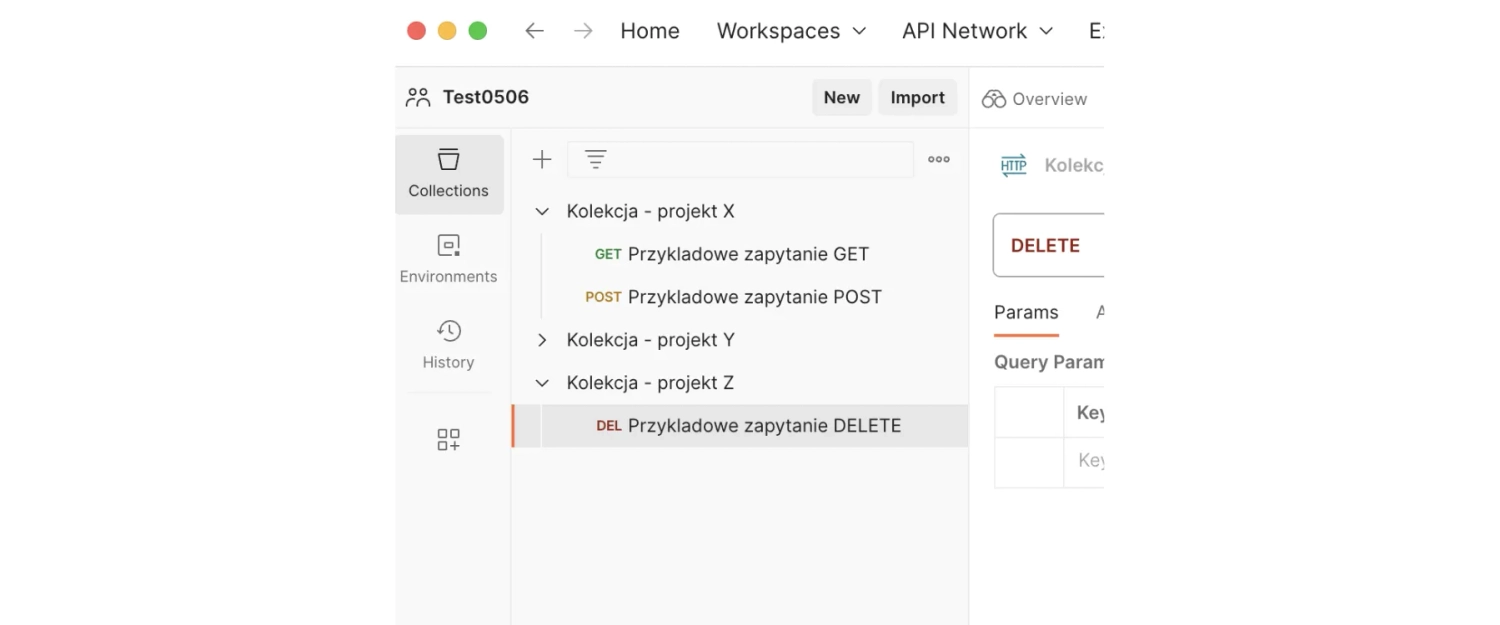
- Query collections- Postman allows you to group queries into collections, making it easier to organize and execute test suites. You can create sequential or parallel tests that test different aspects of the API.
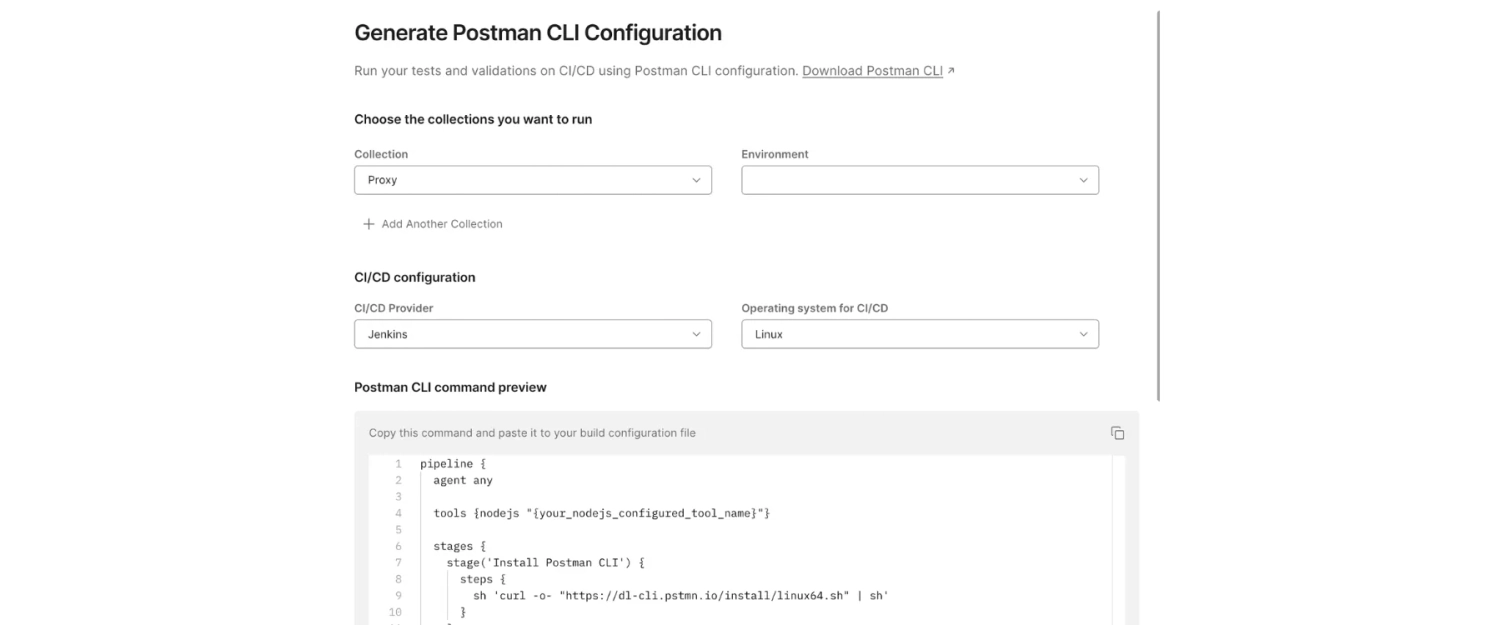
- Integration with CI/CD- Postman is integrated with popular CI/CD tools such as Jenkins and TeamCity. You can automatically run API test suites as part of the CI/CD process and receive reports on test results.
Cypress
Cypress, too, can be used for API testing, although Cypress is mainly known as a user interface (UI) testing tool.
Cypress offers a set of commands that allow you to send HTTP requests, analyze responses and verify API data. To start testing, you can use thecy.request()
command to send HTTP requests to the API and verify the responses. You can also use other Cypress features, such as assertions, to verify the expected data in the API response:
cy.request('GET', '/api/cars/1').then((response) => { expect(response.status).to.equal(200); expect(response.body.name).to.equal('Opel Astra'); });
Postman allows you to use more elaborate methods, such as contains()
, to verify that the returned response element has a given type (in the example below, we check that it is an array) and that it contains a specific element*(BMW X3*):
cy.request('GET', '/api/cars').then((response) => { expect(response.body).to.be.an('array').that.contains('BMW X3'); });
Cypress will be particularly useful when you want to run end-to-end tests that involve both GUI user interface interactions and API communication. Cypress allows you to integrate UI tests with API tests, which makes it possible to verify the correctness of the application on multiple levels and practically perform E2E tests.
Choosing the right tool
In conclusion, for the most popular type of API on the market today, i.e. REST API, the most popular tool for writing automated tests is Postman, which, in addition to having a relatively user-friendly interface and numerous facilities for the tester, also offers very advanced capabilities when it comes to automation. Compared to Postman, when it comes solely to API testing, Cypress is a rather niche tool, which, however, will work great in scenarios where we are testing comprehensively, i.e. API and UI.
If you are interested in the article, we encourage you to check out the training: